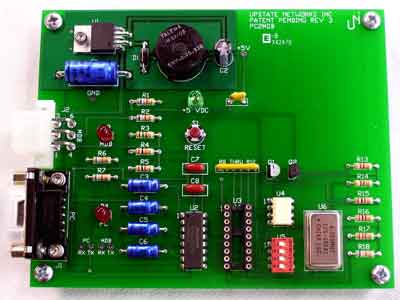
The PC2USD™
PC2USD is a
hardware interface with software and source code that allows a programmer to
control the spirals on a series of vending machines. Dispenses products based
on PC serial port commands. By using the Universal Satellite Device feature of
the MDB protocol, the PC can now dispense by row and column in any USD MDB
equipped vending machine
Example for a
Universal Satellite Device
PC2USD
Screen Shot
Software
and source code (VB 6.0) are included with the PC2MDB
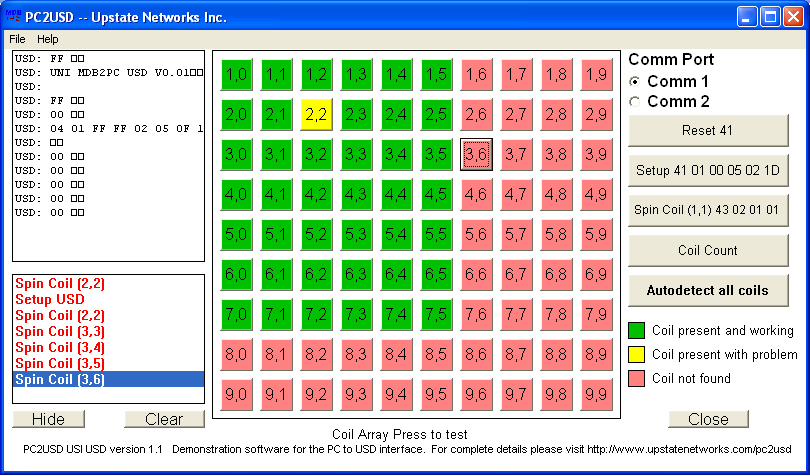
Power requirements
24 to
35 Vdc
90 ma Typical
300 ma Maximum
Environmental
Operating Temp 32°F to 158°F
0°C to 70°C
Storage Temp -22°F to 165°F
-30°C to 74°C
Relative Humidity 5% to 95% Non-condensing
Physical Weight
< 1 lb
Physical Dimensions
Length 4.0 inches Width 3.0 inches Height 1.1 inches
Connector Info
PWR
24-35V Pin 2 +24Vdc Nominal
Pin 4
Ground
MDB Pin
1 +24Vdc Nominal
Pin 2
Ground
Pin 3 N/C
Pin 4 MDB Receive Data
Pin 5 MDB Transmit Data
Pin 6 Common
RS-232 Pin 1 N/C
Pin 2 PC Transmit Data
Pin 3 PC Receive Data
Pin 4 DTR
Pin 5 Ground
Pin 6 N/C
Pin 7 N/C
Pin 8 CTS
Pin 9 N/C
LED/Jumper Designations
D2 +5Vdc
D3 MDB Activity
D4 PC Activity
D6 Pulse Output Activity
Switch 1: Mode Select 1
Switch 2: Mode Select 2
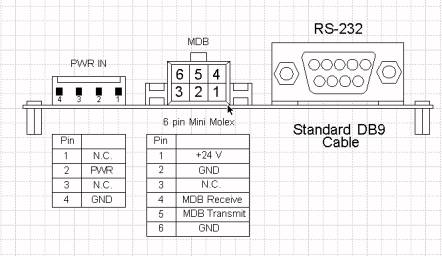
It is time to install the
PC2USD™ itself and move on to the testing phase.
Installation is relatively simple; there are only three connections that must be
made for full functioning of the device. There are
connectors on the edge of the board. One cable plugs into a
24VDC power supply. The 6-pin Molex connects to the VMC. The
final connector is a DB-9 and connects into the back of the computer.
There should be an open port on the back of the computer labeled
“SERIAL2” or “COM2.”
When the master/VMC has data to send we check
the mode bit to differentiate between ADDRESS bytes and DATA bytes. The upper
five bits (MSB) of the Address Byte are used for addressing. The lower three
bits of the Address Byte contain peripheral specific commands. This will allow
up to eight instructions to be embedded in the first byte of block.
The PC2USD sends information generated by the
VMC device directly to the PC via RS-232 serial communication. The PC receives
Data from the PC2MDB in ASCII and transmits in Binary. It responds to polls
issued by the VMC. PC2USD will ACK only the polls, and commands issued to
correct addresses. The PC2USD then forwards the commands to the PC. Once the
data has been processed, the PC sends back another set of instructions to the
PC2USD, which forwards these instructions to the VMC only when desired
poll/polls have been received. The information sent to the PC is send as bytes
in hexadecimal. The first byte sent is the device ID. For example 30 XX means
that a bill validator has sent information. Whereas 08 XX means that a coin
mechanism has sent data. Consult your manual for commands specific to your MDB
device. We have included command sets for various MDB devices in this
document. Please note that all examples of source code are written in MS Visual
Basic 6.0
PC2USD Software Communication
Use an interrupt driven comm event on the
appropriate port. Settings are 9600-8-1-None. Set the receive threshold to 1.
Empty the contents of the receive buffer as soon as there is at least one
character ready. See the sample source code provided on CD-ROM for an example.
******************************************************************
'Receive
******************************************************************
Case comEvReceive 'Received RThreshold # of
Chars
Do While MSComm1.InBufferCount > 0
For ii = 1 To
500000 'Added this delay to allow the input buffer to read
Next ii 'entire contents. Approx
50mSec delay
MDB_Input$ = MSComm1.Input
MDBData.AddItem
"USD: " & MDB_Input$
smdb10$ = Mid$(MDB_Input$, 1,
14) '#10 copies the input string
keeping the original.
smdb11$ = Mid$(smdb10$, 1, 2) '#11 determines what the
response from USD, based on NAMA MDB chapter 9
smdb12$ = Mid$(smdb10$, 4, 5) '#12 is the row and column
smdb14$ = Mid$(smdb10$, 13, 2) '#14 is the USD resoponse
code.
Select Case smdb11$
Case ("08")
'response after a status request, NAMA MDB chapter 9.8
Select Case smdb12$
Case ("01 00") 'row and
column
Select Case smdb14$
'following strings- error
codes
'00 = coil is working and no errors
'01 = coil selection sold out
'02 = motor is jammed
'04 = motor not detected
'05 = invalid
Case ("00")
Command15.BackColor =
QBColor(10) 'green- OK
Case ("01")
Command15.BackColor =QBColor(14) 'yellow- error
Case ("02")
Command15.BackColor = QBColor(14)
Case ("04")
Command15.BackColor =QBColor(12) 'red- don't use
Case ("05")
Command15.BackColor = QBColor(12)
End Select
Use an interrupt driven comm event on the
appropriate port. Settings are 9600-8-1-None. Set the transmit threshold to 1.
Send the address of the device followed by the command parameters.
********************************************************************
'TRANSMIT
********************************************************************
Private Sub Command16_Click()
'Here is an example of a typical command
concatenated using the '&'
'In this case it is the command to spin coil row 2, column 0
MDB_output_string = Chr$(&H43) & Chr$(2)
& Chr$(2) & Chr$(&H0)
'Set DTR high telling the PC2MDB
that we wish to transmit
MSComm1.DTREnable = True 'start
handshaking
'Send detail to the list box so the
user sees something
lstActionLog.AddItem "Spin Coil
(2,0)"
lstActionLog.ListIndex =
lstActionLog.ListCount - 1
Exit Sub
End Sub
Select Case MSComm1.CommEvent
Case comEvCTS 'Change in the CTS line
'this is the output routine for
transmitting outbuffersize set to 34 bytes
MSComm1.Output = MDB_output_string
For ii = 1 To 500000 'Added this delay
to allow the output buffer to clear Next ii 'Transmit entire
contents. 50mSec delay
MSComm1.DTREnable = False
When the PC has data to send to a slave device
the following is required for sending this data:
- Send DTR high (DTR.Enabled=TRUE)
- On Change in CTS line (generated by
the VMC) set output buffer=string to be transmitted
- Place contents of string in output
buffer and transmit
- Set DTR low after transmitting entire
string
Notes: Place the string to be transmitted in
a temporary variable and then place the temporary variable in the output buffer.
**********************************************************************
'HANDSHAKING
**********************************************************************
Since
we have the contents to be transmitted in a temporary string (MDB_output_string)
and we ‘have set DTR high (comInterface.DTREnable = True) we now wait for a
comm. Event to be generated ‘namely a change in the CTS line
:
Case comEvCTS
'Change in the CTS line
'this is the output routine for
transmitting
'outbuffersize set to 32 bytes
comInterface.Output = MDB_output_string
'END transmit one character on change of CTS line
‘At this point the
data has been sent to the output buffer of the serial port UART.
By setting ‘the Send Threshold to 1 we fire a comm. Send event when the
entire contents of the output buffer ‘have been sent and set DTR low to indicate
that we have completed transmission. For example(4)
Case
comEvSend 'There are SThreshold number of characters in
thetransmit buffer
comInterface.DTREnable = True
‘****************************************************************
|